Parameters in the OpenAPI 3.1 specification can be defined in two ways using a JSON schema; either by using a media type object, which is useful when the parameter is complex to describe, or by using styles, which is more common in simple scenarios, which often is the case with HTTP parameters.
Let’s have a look at the styles defined and where they fit into a HTTP request.
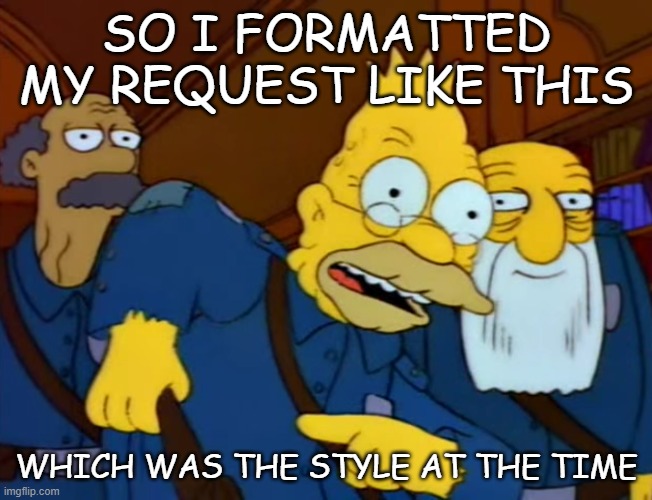
Path Parameters
Path parameters are parameters defined in a path, for example id
in the path /user/{id}
. Path parameters can be described using label, matrix or simple styles, which are all defined by RFC6570, URI Template.
Here are some examples using the JSON primitive value 1
for a parameter named id
:
- Simple:
/user/1
- Matrix:
/user/;id=1
- Label:
/user/.1
It’s also possible to describe arrays. Using the same parameter as above with two JSON primitive values, 1
and 2
, it get’s serialized as:
- Simple:
/users/1,2
- Matrix:
/user/;id=1,2
- Label:
/user/.1.2
Given a JSON object for a parameter named user
with the value, { "id": 1, "name": "foo" }
, it becomes:
- Simple:
/user/id,1,name,foo
- Matrix:
/user/;user=id,1,name,foo
- Label:
/user/.id.1.name.foo
The explode
modifier can be used to enforce composite values (name/value pairs). For primitive values this has no effect, neither for label and simple arrays. With the above examples and styles where explode has effect, here’s the equivalent:
Arrays
- Matrix:
/user/;id=1;id=2
Objects
- Simple:
/user/id=1,name=foo
- Matrix:
/user/;id=1;name=foo
- Label:
/user/.id=1.name=foo
Query Parameters
Query parameters can be described with form, space delimited, pipe delimited or deep object styles. The form style is defined by RFC6570, the rest are defined by OpenAPI.
Primitives
Using the example from path parameters, a serialized user, ?user={user}
, or user id, ?id={id}
, defined as a query parameter value would look like:
- Form:
id=1
Note that the examples doesn’t describe any primitive values for pipe and space delimited styles, even though they are quite similar to the simple style.
Arrays
- Form:
id=1,2
- SpaceDelimited:
id=1%202
- PipeDelimited:
id=1|2
Objects
- Form:
user=id,1,name,foo
- SpaceDelimited:
user=id%201%20name%20foo
- PipeDelimited:
user=id|1|name|foo
Note that the examples lack the parameter name for array and objects, this has been corrected in 3.1.1.
Not defining explode for deepObject style is not applicable, and like path styles, explode doesn’t have effect on primitive values.
Exploded pipe and space delimited parameters are not described in the example, even though they are similar to form. Do note though that neither of them would be possible to parse, as the parameter name cannot be inferred.
With all this in mind here are the respective explode examples:
Arrays
- Form:
id=1&id=2
- SpaceDelimited:
id=1%202
- PipeDelimited:
id=1|2
Objects
- Form:
id=1&name=foo
- SpaceDelimited:
id=1%20name=foo
- PipeDelimited:
id=1|name=foo
- DeepObject:
user[id]=1&user[name]=foo
Header Parameters
Header parameter can only be described using the simple style.
Given the header user: {user}
and id: {id}
, a respective header parameter value with simple style would look like:
Primitives
- Simple:
1
Arrays
- Simple:
1,2
Objects
- Simple:
id,1,name,foo
Similar to the other parameters described, explode with primitive values have no effect, neither for arrays. For objects it would look like:
- Simple:
id=1,name=foo
Cookie Parameters
A cookie parameter can only be described with form style, and is represented in a similar way as query parameters. Using the example Cookie: id={id}
and Cookie: user={user}
a cookie parameter value would look like:
Primitives
- Form:
id=1
Arrays
- Form:
id=1,2
Objects
- Form:
user=id,1,name,foo
Similar to the other parameters described, explode with primitive values have no effect. For arrays and objects it looks like:
Arrays
- Form:
id=1&id=2
Objects
- Form:
id=1&name=foo
Note that exploded objects for cookie parameters have the same problem as query parameters; the parameter name cannot be inferred.
Object Complexity
Theoretically an object can have an endless deep property structure, where each property are objects that also have properties that are objects and so on. RFC6570 nor OpenAPI 3.1 defines how deep a structure can be, but it would be difficult to define array items and object properties as objects in most styles.
As OpenAPI provides media type objects as a complement for complex parameters, it’s advisable to use those instead in such scenarios.
OpenAPI.ParameterStyleParsers
To support parsing and serialization of style defined parameters, I’ve created a .NET library, OpenAPI.ParameterStyleParsers. It parses style serialized parameters into the corresponding JSON instance and vice versa. It supports all examples defined in the OpenAPI 3.1 specification, corrected according to the inconsistencies described earlier. It only supports arrays with primitive item values and objects with primitive property values, for more complex scenarios use media type objects. The JSON instance type the parameter get’s parsed according to is determined by the schema type
keyword. If no type information is defined it falls back on a best effort guess based on the parameter style.