I while ago I wrote about how to detect breaking changes in .NET using Microsoft.DotNet.ApiCompat. Since then ApiCompat has moved into the .NET SDK.
What has Changed?
Since ApiCompat now is part of the .NET SDK, the Arcade package feed doesn’t need to be referenced anymore.
<PropertyGroup>
<RestoreAdditionalProjectSources>
https://pkgs.dev.azure.com/dnceng/public/_packaging/dotnet-eng/nuget/v3/index.json;
</RestoreAdditionalProjectSources>
</PropertyGroup>
The package reference can also be removed.
<ItemGroup>
<PackageReference Include="Microsoft.DotNet.ApiCompat" Version="7.0.0-beta.22115.2" PrivateAssets="All" />
</ItemGroup>
In order to continue running assembly validation from MSBuild, install the Microsoft.DotNet.ApiCompat.Task.
<ItemGroup>
<PackageReference Include="Microsoft.DotNet.ApiCompat.Task" Version="8.0.404" PrivateAssets="all" IsImplicitlyDefined="true" />
</ItemGroup>
Enable Assembly validation.
<PropertyGroup>
<ApiCompatValidateAssemblies>true</ApiCompatValidateAssemblies>
</PropertyGroup>
The contract assembly reference directive has changed, so the old directive needs to be replaced.
<ItemGroup>
<ResolvedMatchingContract Include="LastMajorVersionBinary/lib/$(TargetFramework)/$(AssemblyName).dll" />
</ItemGroup>
<PropertyGroup>
<ApiCompatContractAssembly> LastMajorVersionBinary/lib/$(TargetFramework)/$(AssemblyName).dll
</ApiCompatContractAssembly>
</PropertyGroup>
The property controlling suppressing breaking changes, BaselineAllAPICompatError
, has changed to ApiCompatGenerateSuppressionFile
.
<PropertyGroup>
<BaselineAllAPICompatError>false</BaselineAllAPICompatError>
<ApiCompatGenerateSuppressionFile>false </ApiCompatGenerateSuppressionFile>
</PropertyGroup>
That’s it, your good to go!
Compatibility Baseline / Suppression directives
Previously the suppression file, ApiCompatBaseline.txt
, contained text directives describing suppressed compatibility issues.
Compat issues with assembly Kafka.Protocol:
TypesMustExist : Type 'Kafka.Protocol.ConsumerGroupHeartbeatRequest.Assignor' does not exist in the implementation but it does exist in the contract.
This format has changed to an XML based format, written by default to a file called CompatibilitySuppressions.xml
.
<?xml version="1.0" encoding="utf-8"?>
<Suppressions xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Suppression>
<DiagnosticId>CP0001</DiagnosticId>
<Target> T:Kafka.Protocol.CreateTopicsRequest.CreatableTopic.CreateableTopicConfig
</Target>
<Left>
LastMajorVersionBinary/lib/netstandard2.1/Kafka.Protocol.dll
</Left>
<Right>obj\Debug\netstandard2.1\Kafka.Protocol.dll</Right>
</Suppression>
</Suppressions>
This format is more verbose than the old format, a bit more difficult to read from a human perspective if you ask me. The description of the various DiagnosticIds
can be found in this list.
Path Separator Mismatches
Looking at the suppression example, you might notice that the suppressions contain references to the compared assembly and the baseline contract. It’s not a coincidence that the path separators differs between the references to the contract assembly and the assembly being compared. The Left reference is a templated copy of the ApiCompatContractAssembly
directive using OS agnostic forward slashes, but the Right directive is generated by ApiCompat and it is not OS agnostic, hence the backslash path separators generated when executing under Windows. If ApiCompat is executed under Linux it would generate front slash path separators.
You might also notice that the reference to the assembly being compared contains the build configuration name. This might not match the build configuration name used during a build pipeline for example (Debug vs Release).
Both these differences in path reference will make ApiCompat ignore the suppressions when not matched. There is no documentation on how to consolidate these, but fortunately there are a couple of somewhat hidden transformation directives which can help control how these paths are formatted.
<PropertyGroup>
<_ApiCompatCaptureGroupPattern>
.+%5C$([System.IO.Path]::DirectorySeparatorChar)(.+)%5C$([System.IO.Path]::DirectorySeparatorChar)(.+)
</_ApiCompatCaptureGroupPattern>
</PropertyGroup>
<ItemGroup>
<!-- Make sure the Right suppression directive is OS-agnostic and disregards configuration -->
<ApiCompatRightAssembliesTransformationPattern Include="$(_ApiCompatCaptureGroupPattern)" ReplacementString="obj/$1/$2" />
</ItemGroup>
The _ApiCompatCaptureGroupPattern
regex directive captures path segment groups which can be used in the ApiCompatRightAssembliesTransformationPattern
directive to rewrite the assembly reference path to something that is compatible to both Linux and Windows, and removes the build configuration segment.
Using this will cause the Right
directive to change accordingly.
<?xml version="1.0" encoding="utf-8"?>
<Suppressions xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Suppression>
<DiagnosticId>CP0001</DiagnosticId>
<Target>
T:Kafka.Protocol.CreateTopicsRequest.CreatableTopic.CreateableTopicConfig
</Target>
<Left>
LastMajorVersionBinary/lib/netstandard2.1/Kafka.Protocol.dll
</Left>
<Right>obj/netstandard2.1/Kafka.Protocol.dll</Right>
</Suppression>
</Suppressions>
There is a similar directive for the Left
directive named ApiCompatLeftAssembliesTransformationPattern
.
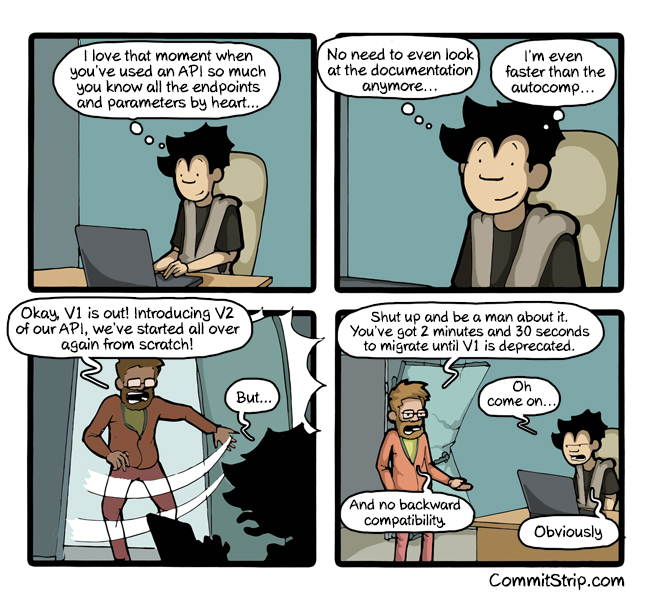